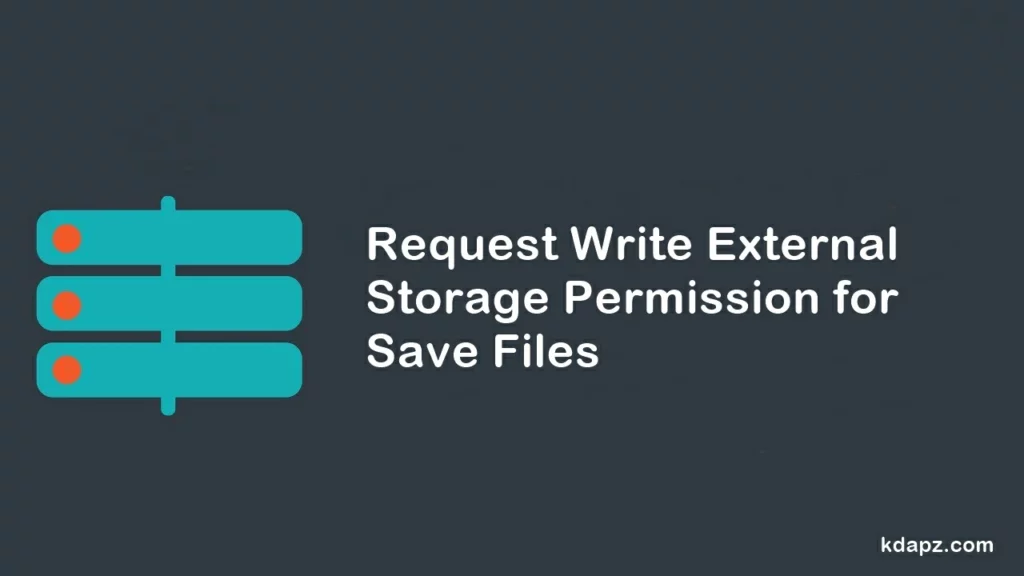
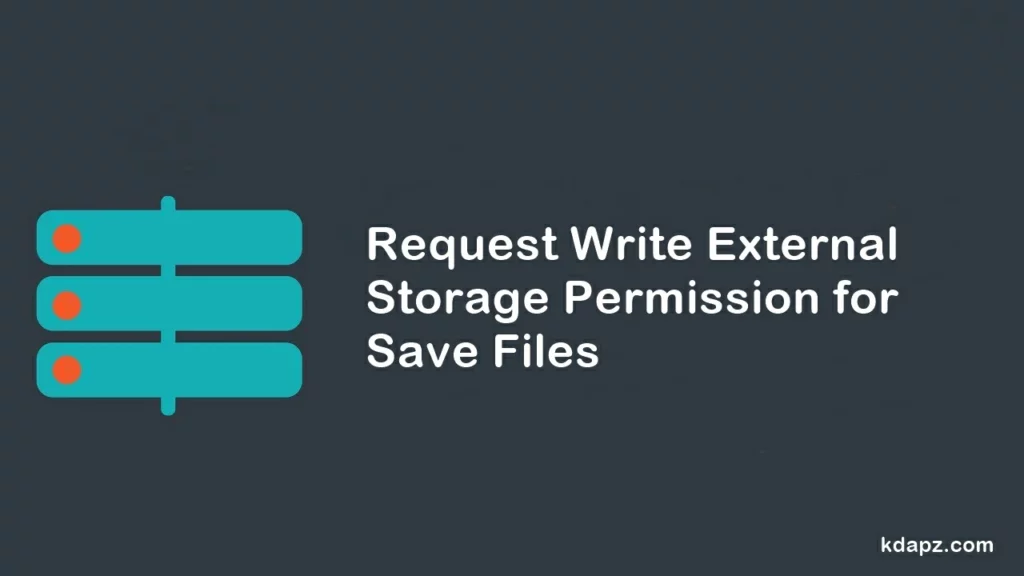
How to Request Write External Storage Permission for Save Files
In this article, we will explain how to add a download manager with a download dialog message and How to request write external storage permission in the Android WebView app. And also we will show you how to require permission from the user. To do that we use 3 steps.
- Download Manager with dialog Message
- Request permissions
- If the user denied the permission show permission required message
Request permissions
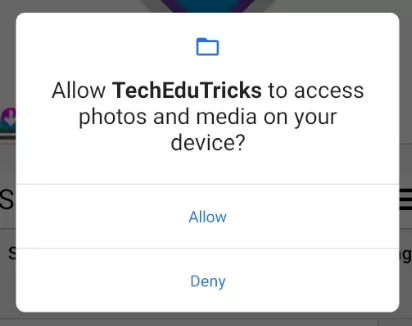
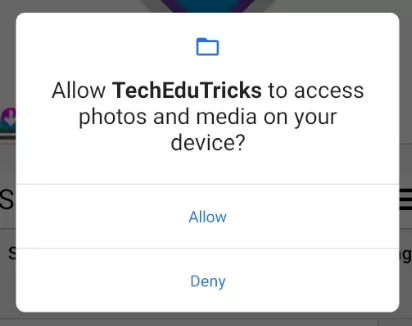
Download Manager with dialog Message
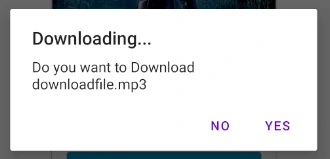
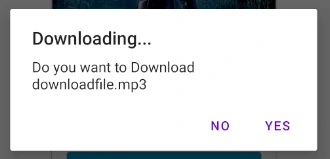
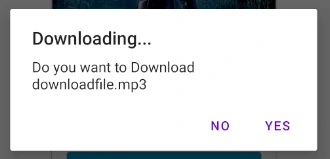
If the user denied the permission show permission required message
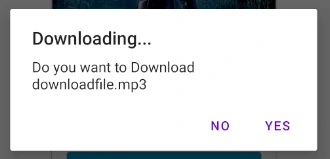
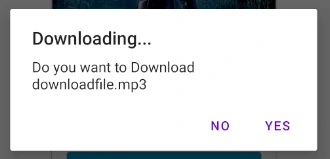
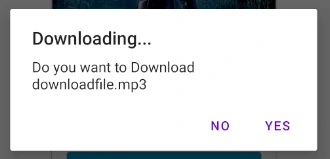
This is our 9 lesson. If you didn’t read earlier articles, please use these links to read them.
Add bellow code to your MainActivity.java inside AppCompatActivity before the onCreate method.
private final static int MY_PERMISSION_REQUEST_CODE = 1; private Activity mActivity;
Now add these codes inside the onCreate method.
Context mContext = getApplicationContext(); mActivity = MainActivity.this;
In webSettings add these,
webSettings.setAllowFileAccess(true); webSettings.setAllowContentAccess(true);
Request Write External Storage Permission for Save Files
To check permission add this. (If shows an error don’t think about that)
checkPermission();
Now let’s setDownloadListner
myWebView.setDownloadListener(new DownloadListener() { @Override public void onDownloadStart(String url, String userAgent, String contentDisposition, String mimetype, long contentLength) { if (Build.VERSION.SDK_INT>=Build.VERSION_CODES.M){ if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_GRANTED){ DownloadDialog(url,userAgent,contentDisposition,mimetype); } else{ ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1); } } else { DownloadDialog(url,userAgent,contentDisposition,mimetype); } } }); }
Now let’s make Download Manager with DownloadDialog. The Android java code below can be used to download any type of file to your Android device’s storage with the file’s original name, be it a .mp3, mp4, jpg, or pdf, the code will download any file that is downloadable.
public void DownloadDialog(final String url, final String UserAgent , String contentDisposition, String mimetype){ final String filename = URLUtil.guessFileName(url,contentDisposition,mimetype); AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Downloading...") .setMessage("Do you want to Download"+' '+" "+filename+""+' ') .setPositiveButton("Yes", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { DownloadManager.Request request = new DownloadManager.Request(Uri.parse(url)); String cookie = CookieManager.getInstance().getCookie(url); request.addRequestHeader("Cookie",cookie); request.addRequestHeader("User-Agent", UserAgent); request.allowScanningByMediaScanner(); request.setNotificationVisibility(DownloadManager.Request.VISIBILITY_VISIBLE_NOTIFY_COMPLETED); DownloadManager manager = (DownloadManager)getSystemService(DOWNLOAD_SERVICE); request.setDestinationInExternalPublicDir(Environment.DIRECTORY_DOWNLOADS,filename); manager.enqueue(request); } }) .setNegativeButton("No", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { dialogInterface.cancel(); } }) .show(); }
Now we are going to check permissions.
protected void checkPermission() { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) { if (shouldShowRequestPermissionRationale(Manifest.permission.WRITE_EXTERNAL_STORAGE)) { //show alert dialog AlertDialog.Builder builder = new AlertDialog.Builder(mActivity); builder.setMessage("Write External Storage Permission is Required"); builder.setTitle("Please Grant Permission"); builder.setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_REQUEST_CODE ); } }); builder.setNeutralButton("Cancel", null); AlertDialog dialog = builder.create(); dialog.show(); } else { //request permission ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_REQUEST_CODE ); } } } }
The final thing is you have to add user permissions code in AndroidManifest.xml.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
Here is the full code in MainActivity.java (remember to change the domain)
package com.techedutricks.techedutricks; import androidx.annotation.NonNull; import androidx.appcompat.app.AppCompatActivity; import androidx.core.app.ActivityCompat; import androidx.core.content.ContextCompat; import android.Manifest; import android.annotation.SuppressLint; import android.app.Activity; import android.app.AlertDialog; import android.app.DownloadManager; import android.content.BroadcastReceiver; import android.content.Context; import android.content.DialogInterface; import android.content.Intent; import android.content.IntentFilter; import android.content.pm.PackageManager; import android.net.Uri; import android.os.Build; import android.os.Bundle; import android.os.Environment; import android.os.Handler; import android.view.Gravity; import android.view.View; import android.webkit.CookieManager; import android.webkit.DownloadListener; import android.webkit.URLUtil; import android.webkit.WebSettings; import android.webkit.WebView; import android.webkit.WebViewClient; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private final static int MY_PERMISSION_REQUEST_CODE = 1; private WebView myWebView; private boolean doubleTap; private Activity mActivity; @SuppressLint("SetJavaScriptEnabled") @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Context mContext = getApplicationContext(); mActivity = MainActivity.this; myWebView = (WebView) findViewById(R.id.webview); WebSettings webSettings=myWebView.getSettings(); webSettings.setJavaScriptEnabled(true); //improve WebView Performance myWebView.getSettings().setRenderPriority(WebSettings.RenderPriority.HIGH); myWebView.getSettings().setCacheMode(WebSettings.LOAD_CACHE_ELSE_NETWORK); myWebView.setScrollBarStyle(View.SCROLLBARS_INSIDE_OVERLAY); webSettings.setDomStorageEnabled(true); webSettings.setLayoutAlgorithm(WebSettings.LayoutAlgorithm.NARROW_COLUMNS); webSettings.setUseWideViewPort(true); webSettings.setSavePassword(true); webSettings.setSaveFormData(true); webSettings.setEnableSmoothTransition(true); webSettings.setAllowFileAccess(true); webSettings.setAllowContentAccess(true); //Load Website myWebView.loadUrl("https://www.sindulanthaya.com"); myWebView.setWebViewClient(new MyWebViewClient()); checkPermission(); myWebView.setDownloadListener(new DownloadListener() { @Override public void onDownloadStart(String url, String userAgent, String contentDisposition, String mimetype, long contentLength) { if (Build.VERSION.SDK_INT>=Build.VERSION_CODES.M){ if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_GRANTED){ DownloadDialog(url,userAgent,contentDisposition,mimetype); } else{ ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1); } } else { DownloadDialog(url,userAgent,contentDisposition,mimetype); } } }); } public void DownloadDialog(final String url, final String UserAgent , String contentDisposition, String mimetype){ final String filename = URLUtil.guessFileName(url,contentDisposition,mimetype); AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Downloading...") .setMessage("Do you want to Download"+' '+" "+filename+""+' ') .setPositiveButton("Yes", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { DownloadManager.Request request = new DownloadManager.Request(Uri.parse(url)); String cookie = CookieManager.getInstance().getCookie(url); request.addRequestHeader("Cookie",cookie); request.addRequestHeader("User-Agent", UserAgent); request.allowScanningByMediaScanner(); request.setNotificationVisibility(DownloadManager.Request.VISIBILITY_VISIBLE_NOTIFY_COMPLETED); DownloadManager manager = (DownloadManager)getSystemService(DOWNLOAD_SERVICE); request.setDestinationInExternalPublicDir(Environment.DIRECTORY_DOWNLOADS,filename); manager.enqueue(request); } }) .setNegativeButton("No", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { dialogInterface.cancel(); } }) .show(); } protected void checkPermission() { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) { if (shouldShowRequestPermissionRationale(Manifest.permission.WRITE_EXTERNAL_STORAGE)) { //show alert dialog AlertDialog.Builder builder = new AlertDialog.Builder(mActivity); builder.setMessage("Write External Storage Permission is Required"); builder.setTitle("Please Grant Permission"); builder.setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_REQUEST_CODE ); } }); builder.setNeutralButton("Cancel", null); AlertDialog dialog = builder.create(); dialog.show(); } else { //request permission ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_REQUEST_CODE ); } } } } private class MyWebViewClient extends WebViewClient{ @Override public boolean shouldOverrideUrlLoading(WebView view,String url){ if (Uri.parse(url).getHost().equals("www.sindulanthaya.com")){ //Content urls open in app return false; }else { //Open external links in external browser or apps Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url)); startActivity(intent); return true; } } } public void onBackPressed(){ if (myWebView.canGoBack()) { myWebView.goBack(); } else if (doubleTap) { super.onBackPressed(); } else { Toast toast = Toast.makeText(this, "Press Again To Exit",Toast.LENGTH_SHORT); toast.setGravity(Gravity.CENTER | Gravity.CENTER_HORIZONTAL, 0,0); toast.show(); doubleTap = true; Handler handler = new Handler(); handler.postDelayed(new Runnable() { @Override public void run() { doubleTap = false; } }, 2000); } } }
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.techedutricks.techedutricks"> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.TechEduTricks"> <activity android:name=".MainActivity" android:hardwareAccelerated="true" > </activity> <activity android:name=".WelcomeScreen"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>