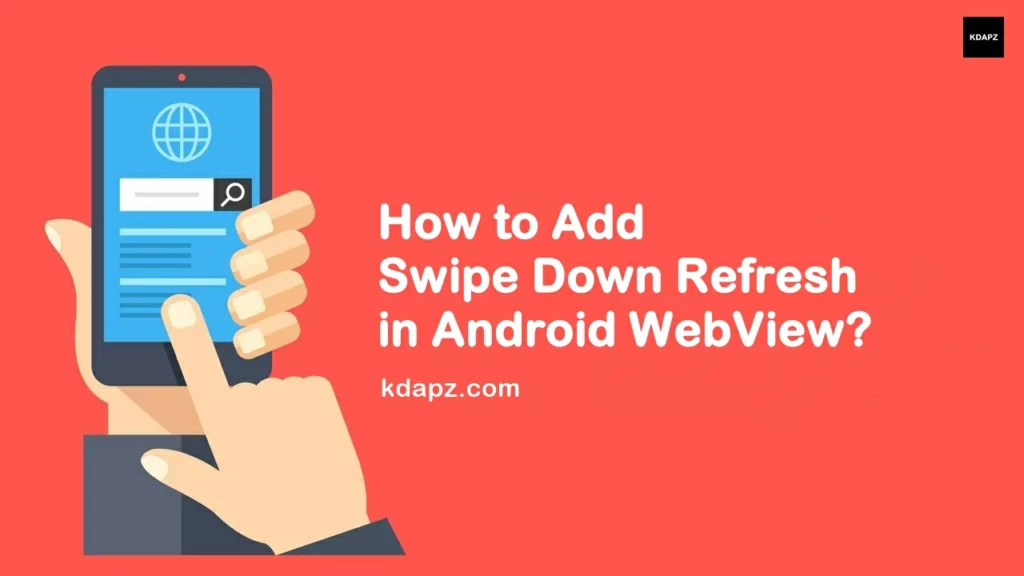
How to Add Swipe Down Refresh in Android WebView?
After this tutorial, your users can refresh your WebView Android app by pulling it down. In this android tutorial, I will tell you how to use the swipe down to refreshing action in android WebView using Android Studio. This source code will help you to refresh the WebView when you swipe down on your android app screen. Refreshing a WebView by swiping down. It will enable the users to refresh the WebView on android.
Android Swipe Down to Refresh a WebView
How to Add Swipe Down Refresh in Android WebView?
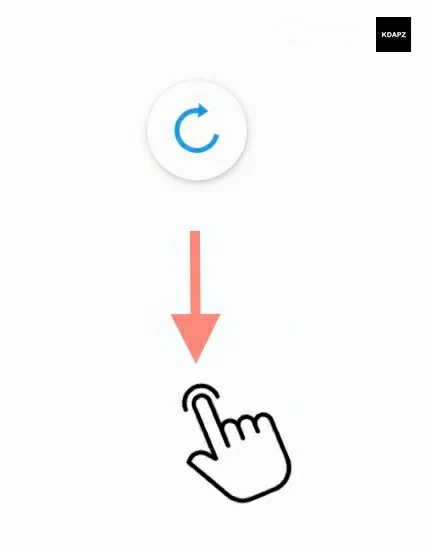
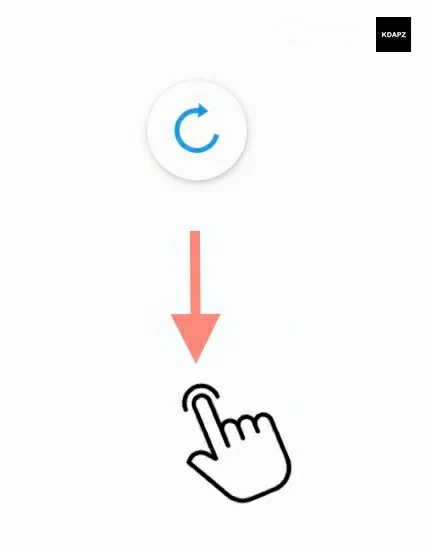
1. Open Your Android Studio Project. (Read previous Articles)
2. Open activity_main_xml and add the following code.
<androidx.swiperefreshlayout.widget.SwipeRefreshLayout android:id="@+id/swipe" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_constraintEnd_toEndOf="parent"> <WebView android:id="@+id/webview" android:layout_width="match_parent" android:layout_height="match_parent" /> </androidx.swiperefreshlayout.widget.SwipeRefreshLayout>
3. Now Open MainActivity.java
in AppCompatActivity and add the following code.
SwipeRefreshLayout swipe;
4. in the Oncreate method add this code.
swipe = (SwipeRefreshLayout) findViewById(R.id.swipe); swipe.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() { @Override public void onRefresh() { myWebView.reload(); } });
5. in WebViewClient add the following code.
myWebView.setWebViewClient(new MyWebViewClient(){ public void onPageFinished(WebView view, String url){ swipe.setRefreshing(false); findViewById(R.id.webview).setVisibility(View.VISIBLE); } });
6. Now set SwipeRefresh true.
swipe.setRefreshing(true);
Here are the full codes…
Main Activity.java
package com.techedutricks.techedutricks; import androidx.annotation.NonNull; import androidx.appcompat.app.AppCompatActivity; import androidx.core.app.ActivityCompat; import androidx.core.content.ContextCompat; import androidx.swiperefreshlayout.widget.SwipeRefreshLayout; import android.Manifest; import android.annotation.SuppressLint; import android.app.Activity; import android.app.AlertDialog; import android.app.DownloadManager; import android.content.BroadcastReceiver; import android.content.Context; import android.content.DialogInterface; import android.content.Intent; import android.content.IntentFilter; import android.content.pm.PackageManager; import android.graphics.Bitmap; import android.net.Uri; import android.os.Build; import android.os.Bundle; import android.os.Environment; import android.os.Handler; import android.view.Gravity; import android.view.View; import android.webkit.CookieManager; import android.webkit.DownloadListener; import android.webkit.URLUtil; import android.webkit.WebChromeClient; import android.webkit.WebSettings; import android.webkit.WebView; import android.webkit.WebViewClient; import android.widget.ProgressBar; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private WebView myWebView; private boolean doubleTap; private final static int MY_PERMISSION_CODE = 1; private Activity mActivity; SwipeRefreshLayout swipe; @SuppressLint("SetJavaScriptEnabled") @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Context mContext = getApplicationContext(); mActivity = MainActivity.this; swipe = (SwipeRefreshLayout) findViewById(R.id.swipe); swipe.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() { @Override public void onRefresh() { myWebView.reload(); } }); myWebView = (WebView) findViewById(R.id.webview); WebSettings webSettings = myWebView.getSettings(); webSettings.setJavaScriptEnabled(true); //improve WebView Performance myWebView.getSettings().setRenderPriority(WebSettings.RenderPriority.HIGH); myWebView.getSettings().setCacheMode(WebSettings.LOAD_CACHE_ELSE_NETWORK); myWebView.setScrollBarStyle(View.SCROLLBARS_INSIDE_OVERLAY); webSettings.setDomStorageEnabled(true); webSettings.setLayoutAlgorithm(WebSettings.LayoutAlgorithm.NARROW_COLUMNS); webSettings.setUseWideViewPort(true); webSettings.setSavePassword(true); webSettings.setSaveFormData(true); webSettings.setEnableSmoothTransition(true); webSettings.setAllowFileAccess(true); webSettings.setAllowContentAccess(true); //Load Website myWebView.setWebViewClient(new MyWebViewClient(){ public void onPageFinished(WebView view, String url){ swipe.setRefreshing(false); findViewById(R.id.webview).setVisibility(View.VISIBLE); } }); //Load Website myWebView.loadUrl("https://www.techedutricks.com"); swipe.setRefreshing(true); checkPermission(); myWebView.setDownloadListener(new DownloadListener() { @Override public void onDownloadStart(String url, String userAgent, String contentDisposition, String mimetype, long contentLength) { if (Build.VERSION.SDK_INT>=Build.VERSION_CODES.M){ if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_GRANTED){ DownloadDialog(url,userAgent,contentDisposition,mimetype); } else { ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1); } } else { DownloadDialog(url,userAgent,contentDisposition,mimetype); } } }); } private void DownloadDialog(String url, String userAgent, String contentDisposition, String mimetype) { final String filename = URLUtil.guessFileName(url,contentDisposition,mimetype); AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Downloading...") .setMessage("Do you want to download"+' '+" "+filename+""+' ') .setPositiveButton("Yes", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { DownloadManager.Request request = new DownloadManager.Request(Uri.parse(url)); String cookie = CookieManager.getInstance().getCookie(url); request.addRequestHeader("Cookie", cookie); request.addRequestHeader("User-Agent", userAgent); request.allowScanningByMediaScanner(); request.setNotificationVisibility(DownloadManager.Request.VISIBILITY_VISIBLE_NOTIFY_COMPLETED); DownloadManager manager = (DownloadManager)getSystemService(DOWNLOAD_SERVICE); request.setDestinationInExternalPublicDir(Environment.DIRECTORY_DOWNLOADS,filename); manager.enqueue(request); } }) .setNegativeButton("No", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { dialogInterface.cancel(); } }) .show(); } private void checkPermission() { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M){ if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED){ if (shouldShowRequestPermissionRationale(Manifest.permission.WRITE_EXTERNAL_STORAGE)){ //show dialog AlertDialog.Builder builder = new AlertDialog.Builder(mActivity); builder.setMessage("Write External Storage Permission is Required"); builder.setTitle("Please Grant Permission"); builder.setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_CODE ); } }); builder.setNegativeButton("Cancel", null); AlertDialog dialog = builder.create(); dialog.show(); } else { //request permission ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_CODE ); } } } } private class MyWebViewClient extends WebViewClient{ @Override public boolean shouldOverrideUrlLoading(WebView view,String url){ if (Uri.parse(url).getHost().equals("techedutricks.com")){ //Content urls open in app return false; }else { //Open external links in external browser or apps Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url)); startActivity(intent); return true; } } } public void onBackPressed(){ if (myWebView.canGoBack()) { myWebView.goBack(); } else if (doubleTap) { super.onBackPressed(); } else { Toast toast = Toast.makeText(this, "Press Again To Exit",Toast.LENGTH_SHORT); toast.setGravity(Gravity.CENTER | Gravity.CENTER_HORIZONTAL, 0,0); toast.show(); doubleTap = true; Handler handler = new Handler(); handler.postDelayed(new Runnable() { @Override public void run() { doubleTap = false; } }, 2000); } } }
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <androidx.swiperefreshlayout.widget.SwipeRefreshLayout android:id="@+id/swipe" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_constraintEnd_toEndOf="parent"> <WebView android:id="@+id/webview" android:layout_width="match_parent" android:layout_height="match_parent" /> </androidx.swiperefreshlayout.widget.SwipeRefreshLayout> </androidx.constraintlayout.widget.ConstraintLayout>
In the Next Article, we will discuss How to show an error page when the user hasn’t internet?