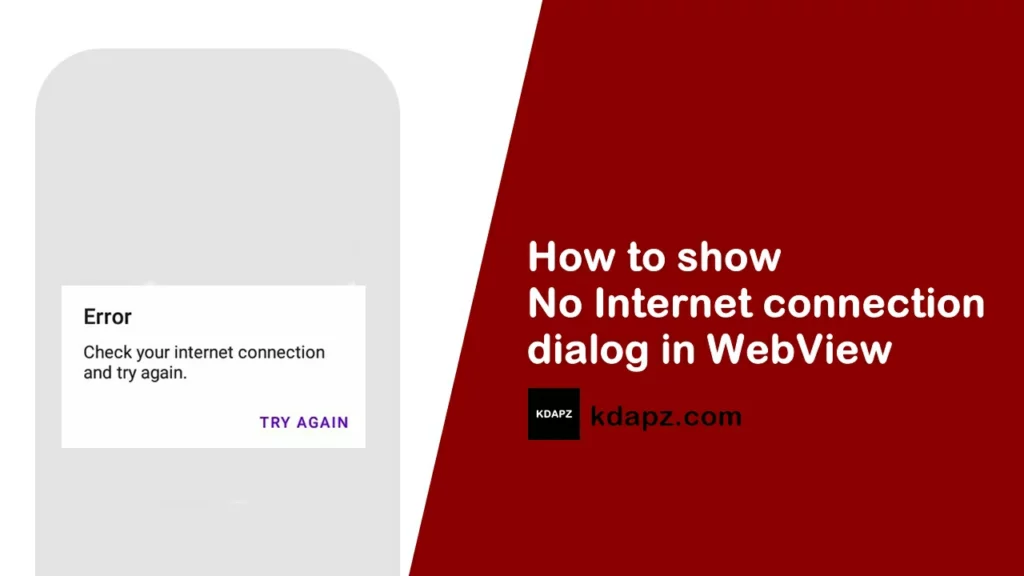
How to show No Internet connection dialog in WebView Android Studio?
Hi Everyone, Let’s continue with our WebView app development series. This is our 11th article. In this Android tutorial lesson, we will show you How to check internet connection and How to show No Internet connection dialog.
Here, I will show you how to fix the ‘WebView webpage not available’ page instead of the ‘no internet connection’ alert box page. And we will add a “Try again” button to reload the web page.
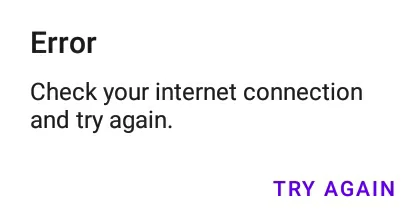
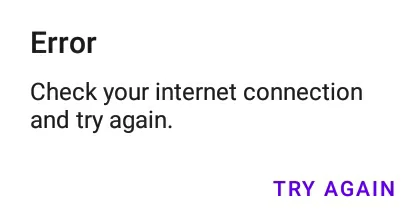
Actually, this is very simple code. You have easily made this problem instead of webpage not available in no internet connection alert box. In the previous post, I explained How to Add Swipe Down Refresh in Android WebView?
How to show No Internet connection dialog in WebView
1. First Open your Android Studio project.
2. Then inside MyWebViewClient add this code.
public void onReceivedError(WebView webView, int errorCode, String description, String failingUrl) { try { webView.stopLoading(); } catch (Exception ignored) { } if (webView.canGoBack()) { webView.goBack(); } webView.loadUrl("#"); AlertDialog alertDialog = new AlertDialog.Builder(MainActivity.this).create(); alertDialog.setTitle("Error"); alertDialog.setMessage("Check your internet connection and try again."); alertDialog.setButton(DialogInterface.BUTTON_POSITIVE, "Try Again", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { finish(); startActivity(getIntent()); } }); alertDialog.show(); super.onReceivedError(webView, errorCode, description, failingUrl); }
Here is the full code (MainActivity.java)
package com.techedutricks.techedutricks; import androidx.annotation.NonNull; import androidx.appcompat.app.AppCompatActivity; import androidx.core.app.ActivityCompat; import androidx.core.content.ContextCompat; import androidx.swiperefreshlayout.widget.SwipeRefreshLayout; import android.Manifest; import android.annotation.SuppressLint; import android.app.Activity; import android.app.AlertDialog; import android.app.DownloadManager; import android.content.BroadcastReceiver; import android.content.Context; import android.content.DialogInterface; import android.content.Intent; import android.content.IntentFilter; import android.content.pm.PackageManager; import android.graphics.Bitmap; import android.net.Uri; import android.os.Build; import android.os.Bundle; import android.os.Environment; import android.os.Handler; import android.view.Gravity; import android.view.View; import android.webkit.CookieManager; import android.webkit.DownloadListener; import android.webkit.URLUtil; import android.webkit.WebChromeClient; import android.webkit.WebSettings; import android.webkit.WebView; import android.webkit.WebViewClient; import android.widget.ProgressBar; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private WebView myWebView; private boolean doubleTap; private final static int MY_PERMISSION_CODE = 1; private Activity mActivity; SwipeRefreshLayout swipe; @SuppressLint("SetJavaScriptEnabled") @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Context mContext = getApplicationContext(); mActivity = MainActivity.this; swipe = (SwipeRefreshLayout) findViewById(R.id.swipe); swipe.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() { @Override public void onRefresh() { myWebView.reload(); } }); myWebView = (WebView) findViewById(R.id.webview); WebSettings webSettings = myWebView.getSettings(); webSettings.setJavaScriptEnabled(true); //improve WebView Performance myWebView.getSettings().setRenderPriority(WebSettings.RenderPriority.HIGH); myWebView.getSettings().setCacheMode(WebSettings.LOAD_CACHE_ELSE_NETWORK); myWebView.setScrollBarStyle(View.SCROLLBARS_INSIDE_OVERLAY); webSettings.setDomStorageEnabled(true); webSettings.setLayoutAlgorithm(WebSettings.LayoutAlgorithm.NARROW_COLUMNS); webSettings.setUseWideViewPort(true); webSettings.setSavePassword(true); webSettings.setSaveFormData(true); webSettings.setEnableSmoothTransition(true); webSettings.setAllowFileAccess(true); webSettings.setAllowContentAccess(true); myWebView.setWebViewClient(new MyWebViewClient() { public void onReceivedError(WebView webView, int errorCode, String description, String failingUrl) { try { webView.stopLoading(); } catch (Exception ignored) { } if (webView.canGoBack()) { webView.goBack(); } webView.loadUrl("#"); AlertDialog alertDialog = new AlertDialog.Builder(MainActivity.this).create(); alertDialog.setTitle("Error"); alertDialog.setMessage("Check your internet connection and try again."); alertDialog.setButton(DialogInterface.BUTTON_POSITIVE, "Try Again", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { finish(); startActivity(getIntent()); } }); alertDialog.show(); super.onReceivedError(webView, errorCode, description, failingUrl); } public void onPageFinished(WebView view, String url){ swipe.setRefreshing(false); findViewById(R.id.webview).setVisibility(View.VISIBLE); } } ); //Load Website myWebView.loadUrl("https://www.techedutricks.com"); swipe.setRefreshing(true); checkPermission(); myWebView.setDownloadListener(new DownloadListener() { @Override public void onDownloadStart(String url, String userAgent, String contentDisposition, String mimetype, long contentLength) { if (Build.VERSION.SDK_INT>=Build.VERSION_CODES.M){ if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_GRANTED){ DownloadDialog(url,userAgent,contentDisposition,mimetype); } else { ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1); } } else { DownloadDialog(url,userAgent,contentDisposition,mimetype); } } }); } private void DownloadDialog(String url, String userAgent, String contentDisposition, String mimetype) { final String filename = URLUtil.guessFileName(url,contentDisposition,mimetype); AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Downloading...") .setMessage("Do you want to download"+' '+" "+filename+""+' ') .setPositiveButton("Yes", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { DownloadManager.Request request = new DownloadManager.Request(Uri.parse(url)); String cookie = CookieManager.getInstance().getCookie(url); request.addRequestHeader("Cookie", cookie); request.addRequestHeader("User-Agent", userAgent); request.allowScanningByMediaScanner(); request.setNotificationVisibility(DownloadManager.Request.VISIBILITY_VISIBLE_NOTIFY_COMPLETED); DownloadManager manager = (DownloadManager)getSystemService(DOWNLOAD_SERVICE); request.setDestinationInExternalPublicDir(Environment.DIRECTORY_DOWNLOADS,filename); manager.enqueue(request); } }) .setNegativeButton("No", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { dialogInterface.cancel(); } }) .show(); } private void checkPermission() { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M){ if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED){ if (shouldShowRequestPermissionRationale(Manifest.permission.WRITE_EXTERNAL_STORAGE)){ //show dialog AlertDialog.Builder builder = new AlertDialog.Builder(mActivity); builder.setMessage("Write External Storage Permission is Required"); builder.setTitle("Please Grant Permission"); builder.setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_CODE ); } }); builder.setNegativeButton("Cancel", null); AlertDialog dialog = builder.create(); dialog.show(); } else { //request permission ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_CODE ); } } } } private class MyWebViewClient extends WebViewClient{ @Override public boolean shouldOverrideUrlLoading(WebView view,String url){ if (Uri.parse(url).getHost().equals("techedutricks.com")){ //Content urls open in app return false; }else { //Open external links in external browser or apps Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url)); startActivity(intent); return true; } } } public void onBackPressed(){ if (myWebView.canGoBack()) { myWebView.goBack(); } else if (doubleTap) { super.onBackPressed(); } else { Toast toast = Toast.makeText(this, "Press Again To Exit",Toast.LENGTH_SHORT); toast.setGravity(Gravity.CENTER | Gravity.BOTTOM, 0,0); toast.show(); doubleTap = true; Handler handler = new Handler(); handler.postDelayed(new Runnable() { @Override public void run() { doubleTap = false; } }, 2000); } } }