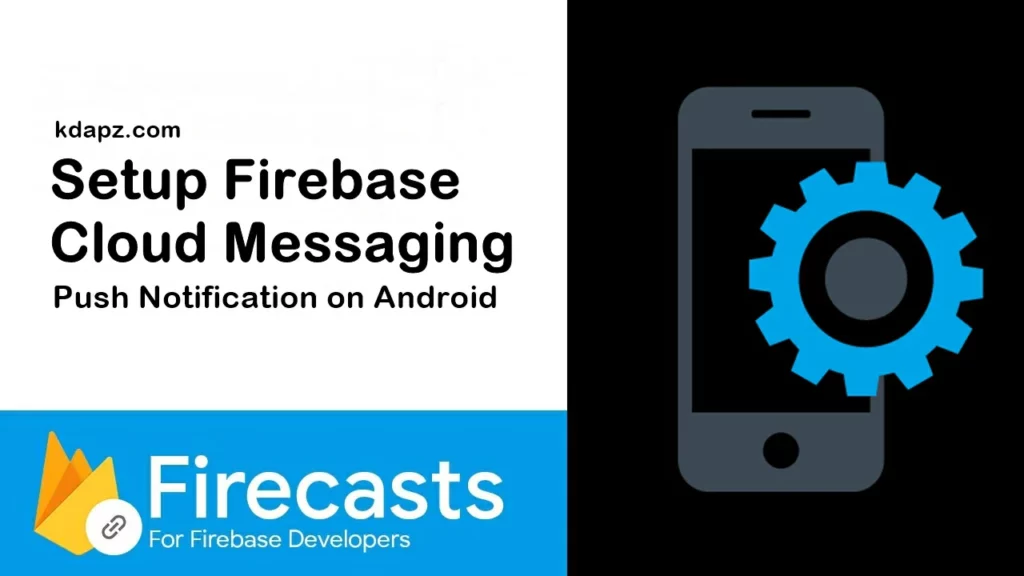
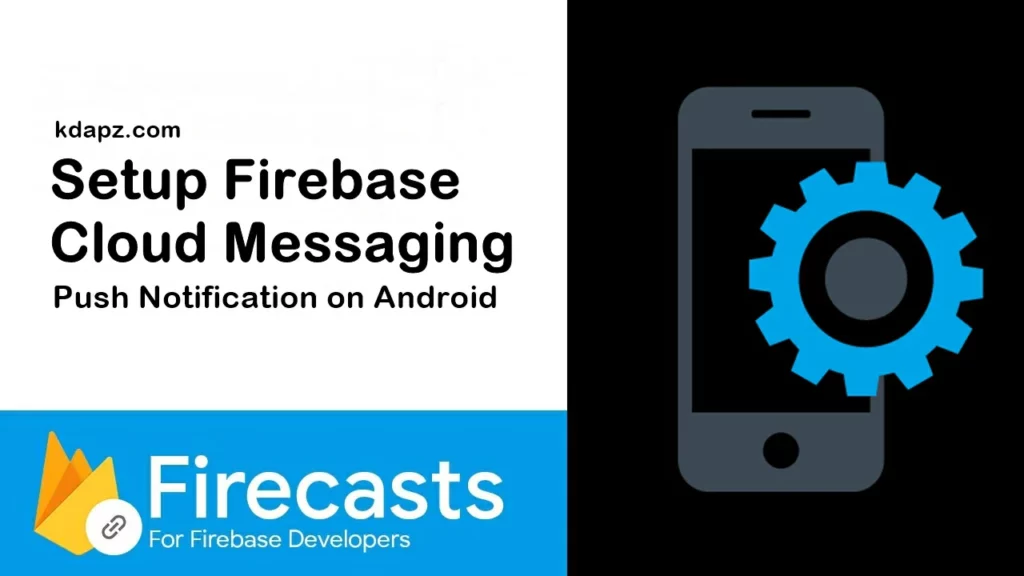
Setup Firebase Cloud Messaging | Push Notification on Android
To send push notifications on your android app you need to connect firebase with the Android Studio project. And also you need to add JSON files and Firebase dependencies.
Sending Push Notifications by Using Firebase Cloud Messaging
Firebase Cloud Messaging is the tool used to send push notifications to a single or a group of devices. Firebase Cloud Messaging: Firebase Cloud Messaging (FCM) is a cross-platform messaging solution that lets you reliably send messages at no cost.
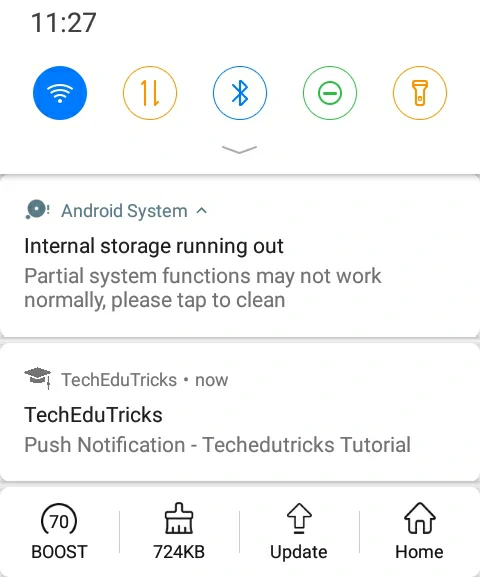
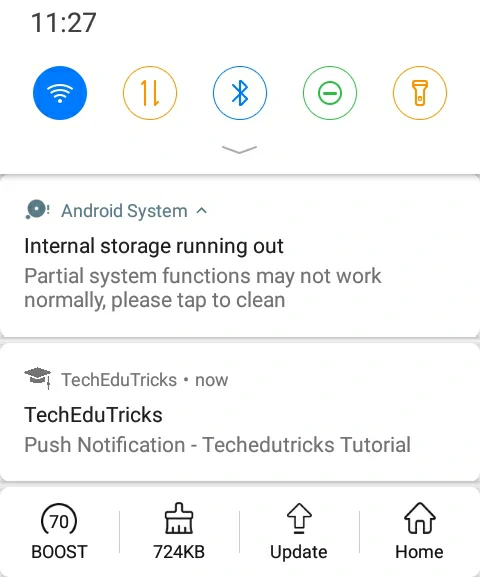
Using FCM, you can notify a client app that a new email or other data is available to sync. You can send notification messages to drive user re-engagement and retention. For use cases such as instant messaging, a message can transfer a payload of up to 4KB to a client app.
Read the “How to Add Firebase to your Android project” Article to connect your Android Studio with Firebase.
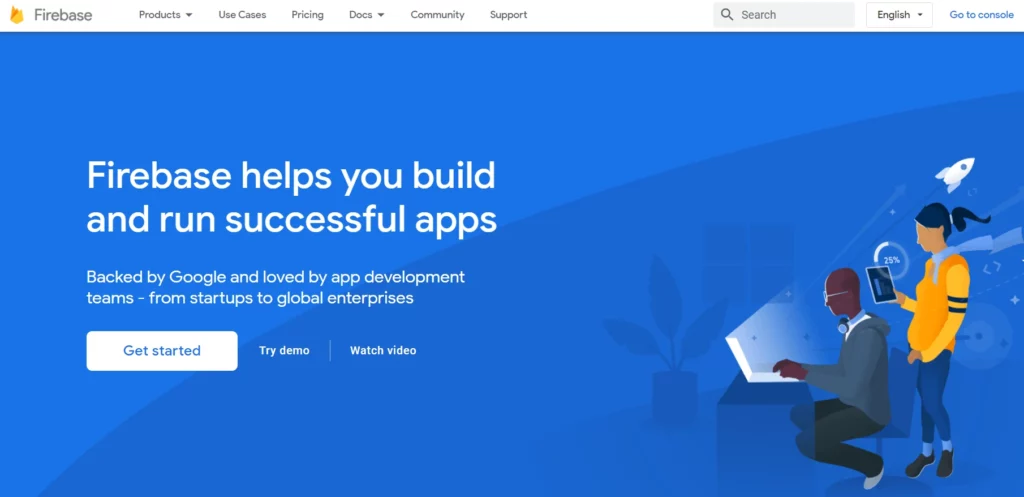
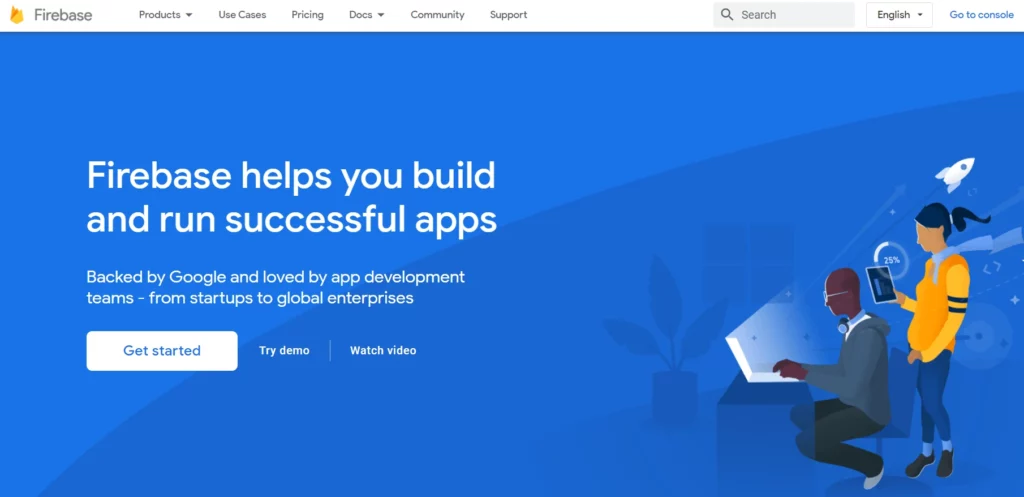
Setup Firebase Cloud Messaging | Push Notification on Android
1. Open Your Android Studio Project
2. in Androidmanifest.xml add the following services and Meta tags.
<service android:name=".MyFirebaseMessagingService"> <intent-filter> <action android:name="com.google.firebase.MESSAGING_EVENT"/> </intent-filter> </service> <meta-data android:name="com.google.firebase.messaging.default_notification_icon" android:resource="@drawable/techedutricks_logo" /> <meta-data android:name="com.google.firebase.messaging.default_notification_color" android:resource="@color/teal_200" />
3. Now create a class using the MyFirebaseMessagingService name. in MyFirebaseMessagingService.java add the following code to receive and customize your notification/message.
public class MyFirebaseMessagingService extends FirebaseMessagingService { @Override public void onMessageReceived(RemoteMessage remoteMessage) { showNotification(remoteMessage.getNotification().getBody()); } private void showNotification(String message) { PendingIntent pi=PendingIntent.getActivity(this,0, new Intent(this,MainActivity.class), 0); Notification notification = new NotificationCompat.Builder(this) .setSmallIcon(R.drawable.techedutricks_logo) .setContentTitle("TechEduTricks") .setContentText(message) .setContentIntent(pi) .setAutoCancel(true) .setDefaults(Notification.FLAG_ONLY_ALERT_ONCE) .setDefaults(Notification.DEFAULT_SOUND) .setDefaults(Notification.DEFAULT_VIBRATE) .build(); NotificationManager notificationManager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE); notificationManager.notify(0, notification); } }
4. Now in your MainActivity.java inside oncreate add the following code.
FirebaseMessaging.getInstance().setAutoInitEnabled(true);
5. Now Send Cloud Message / Push Notification using Firebase Console.
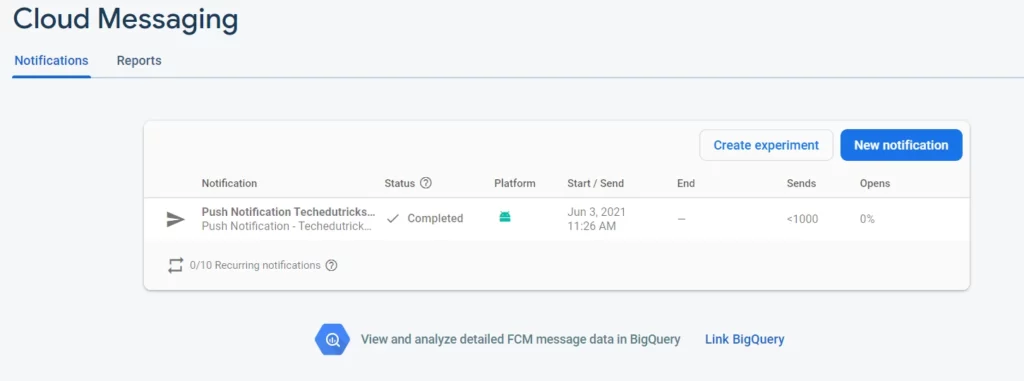
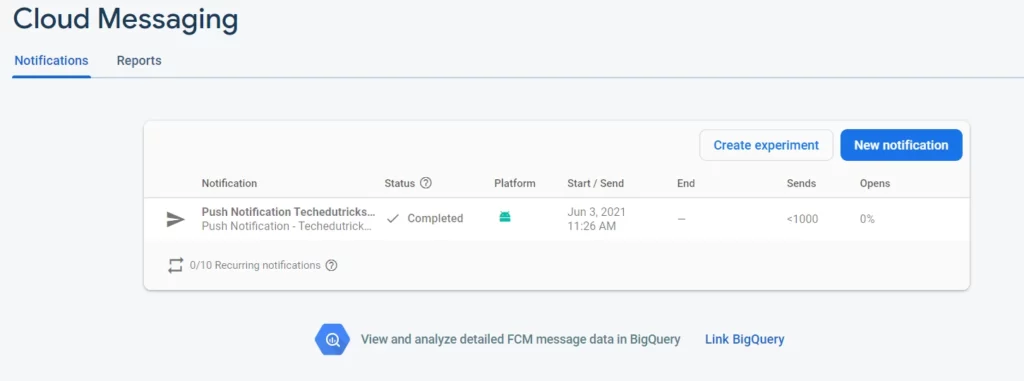
Here are the full codes.
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.techedutricks.techedutricks"> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.TechEduTricks"> <activity android:name=".MainActivity" android:configChanges="keyboard|keyboardHidden|orientation|screenLayout|uiMode|screenSize|smallestScreenSize" android:hardwareAccelerated="true" > </activity> <activity android:name=".WelcomeScreen"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <service android:name=".MyFirebaseMessagingService"> <intent-filter> <action android:name="com.google.firebase.MESSAGING_EVENT"/> </intent-filter> </service> <meta-data android:name="com.google.firebase.messaging.default_notification_icon" android:resource="@drawable/techedutricks_logo" /> <meta-data android:name="com.google.firebase.messaging.default_notification_color" android:resource="@color/teal_200" /> </application> </manifest>
MyFirebaseMessagingService.java
package com.techedutricks.techedutricks; import android.app.Notification; import android.app.NotificationManager; import android.app.PendingIntent; import android.app.Service; import android.content.Intent; import androidx.core.app.NotificationCompat; import androidx.core.app.NotificationManagerCompat; import com.google.firebase.messaging.FirebaseMessagingService; import com.google.firebase.messaging.RemoteMessage; public class MyFirebaseMessagingService extends FirebaseMessagingService { @Override public void onMessageReceived(RemoteMessage remoteMessage) { showNotification(remoteMessage.getNotification().getBody()); } private void showNotification(String message) { PendingIntent pi=PendingIntent.getActivity(this,0, new Intent(this,MainActivity.class), 0); Notification notification = new NotificationCompat.Builder(this) .setSmallIcon(R.drawable.techedutricks_logo) .setContentTitle("TechEduTricks") .setContentText(message) .setContentIntent(pi) .setAutoCancel(true) .setDefaults(Notification.FLAG_ONLY_ALERT_ONCE) .setDefaults(Notification.DEFAULT_SOUND) .setDefaults(Notification.DEFAULT_VIBRATE) .build(); NotificationManager notificationManager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE); notificationManager.notify(0, notification); } }
MainActivity.java
package com.techedutricks.techedutricks; import androidx.annotation.NonNull; import androidx.annotation.RequiresApi; import androidx.appcompat.app.AppCompatActivity; import androidx.core.app.ActivityCompat; import androidx.core.content.ContextCompat; import androidx.swiperefreshlayout.widget.SwipeRefreshLayout; import android.Manifest; import android.annotation.SuppressLint; import android.app.Activity; import android.app.AlertDialog; import android.app.DownloadManager; import android.content.ActivityNotFoundException; import android.content.BroadcastReceiver; import android.content.Context; import android.content.DialogInterface; import android.content.Intent; import android.content.IntentFilter; import android.content.pm.PackageManager; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.net.Uri; import android.os.Build; import android.os.Bundle; import android.os.Environment; import android.os.Handler; import android.view.Gravity; import android.view.View; import android.webkit.CookieManager; import android.webkit.DownloadListener; import android.webkit.URLUtil; import android.webkit.ValueCallback; import android.webkit.WebChromeClient; import android.webkit.WebSettings; import android.webkit.WebView; import android.webkit.WebViewClient; import android.widget.FrameLayout; import android.widget.ProgressBar; import android.widget.Toast; import com.google.firebase.messaging.FirebaseMessaging; public class MainActivity extends AppCompatActivity { private WebView myWebView; private boolean doubleTap; private final static int MY_PERMISSION_CODE = 1; private Activity mActivity; SwipeRefreshLayout swipe; private ValueCallback<Uri> mUploadMessage; public ValueCallback<Uri[]> uploadMessage; public static final int REQUEST_SELECT_FILE = 100; private final static int FILECHOOSER_RESULTCODE = 1; @SuppressLint("SetJavaScriptEnabled") @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Context mContext = getApplicationContext(); mActivity = MainActivity.this; FirebaseMessaging.getInstance().setAutoInitEnabled(true); swipe = (SwipeRefreshLayout) findViewById(R.id.swipe); swipe.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() { @Override public void onRefresh() { myWebView.reload(); } }); myWebView = (WebView) findViewById(R.id.webview); WebSettings webSettings = myWebView.getSettings(); webSettings.setJavaScriptEnabled(true); //improve WebView Performance myWebView.getSettings().setRenderPriority(WebSettings.RenderPriority.HIGH); myWebView.getSettings().setCacheMode(WebSettings.LOAD_CACHE_ELSE_NETWORK); myWebView.setScrollBarStyle(View.SCROLLBARS_INSIDE_OVERLAY); webSettings.setDomStorageEnabled(true); webSettings.setLayoutAlgorithm(WebSettings.LayoutAlgorithm.NARROW_COLUMNS); webSettings.setUseWideViewPort(true); webSettings.setSavePassword(true); webSettings.setSaveFormData(true); webSettings.setEnableSmoothTransition(true); webSettings.setAllowFileAccess(true); webSettings.setAllowContentAccess(true); webSettings.setMediaPlaybackRequiresUserGesture(false); myWebView.setWebViewClient(new MyWebViewClient() { public void onReceivedError(WebView webView, int errorCode, String description, String failingUrl) { try { webView.stopLoading(); } catch (Exception ignored) { } if (webView.canGoBack()) { webView.goBack(); } webView.loadUrl("#"); AlertDialog alertDialog = new AlertDialog.Builder(MainActivity.this).create(); alertDialog.setTitle("Error"); alertDialog.setMessage("Check Your internet Connection And Try Again"); alertDialog.setButton(DialogInterface.BUTTON_POSITIVE, "Try Again", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { finish(); startActivity(getIntent()); } }); alertDialog.show(); super.onReceivedError(webView, errorCode, description, failingUrl); } public void onPageFinished(WebView view, String url) { swipe.setRefreshing(false); findViewById(R.id.webview).setVisibility(View.VISIBLE); } } ); myWebView.setWebChromeClient(new MyWebClient() { // For 3.0+ Devices (Start) // onActivityResult attached before constructor protected void openFileChooser(ValueCallback uploadMsg, String acceptType) { mUploadMessage = uploadMsg; Intent i = new Intent(Intent.ACTION_GET_CONTENT); i.addCategory(Intent.CATEGORY_OPENABLE); i.setType("/*"); startActivityForResult(Intent.createChooser(i, "File Browser"), FILECHOOSER_RESULTCODE); } // For Lollipop 5.0+ Devices @RequiresApi(api = Build.VERSION_CODES.LOLLIPOP) public boolean onShowFileChooser(WebView mWebView, ValueCallback<Uri[]> filePathCallback, FileChooserParams fileChooserParams) { if (uploadMessage != null) { uploadMessage.onReceiveValue(null); uploadMessage = null; } uploadMessage = filePathCallback; Intent intent = fileChooserParams.createIntent(); try { startActivityForResult(intent, REQUEST_SELECT_FILE); } catch (ActivityNotFoundException e) { uploadMessage = null; Toast.makeText(getApplicationContext(), "Cannot Open File Chooser", Toast.LENGTH_LONG).show(); return false; } return true; } //For Android 4.1 only protected void openFileChooser(ValueCallback<Uri> uploadMsg, String acceptType, String capture) { mUploadMessage = uploadMsg; Intent intent = new Intent(Intent.ACTION_GET_CONTENT); intent.addCategory(Intent.CATEGORY_OPENABLE); intent.setType("/*"); startActivityForResult(Intent.createChooser(intent, "File Browser"), FILECHOOSER_RESULTCODE); } protected void openFileChooser(ValueCallback<Uri> uploadMsg) { mUploadMessage = uploadMsg; Intent i = new Intent(Intent.ACTION_GET_CONTENT); i.addCategory(Intent.CATEGORY_OPENABLE); i.setType("/*"); startActivityForResult(Intent.createChooser(i, "File Chooser"), FILECHOOSER_RESULTCODE); } }); //Load Website myWebView.loadUrl("https://www.irmone.com"); swipe.setRefreshing(true); checkPermission(); myWebView.setDownloadListener(new DownloadListener() { @Override public void onDownloadStart(String url, String userAgent, String contentDisposition, String mimetype, long contentLength) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_GRANTED) { DownloadDialog(url, userAgent, contentDisposition, mimetype); } else { ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1); } } else { DownloadDialog(url, userAgent, contentDisposition, mimetype); } } }); } private void DownloadDialog(String url, String userAgent, String contentDisposition, String mimetype) { final String filename = URLUtil.guessFileName(url, contentDisposition, mimetype); AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Downloading...") .setMessage("Do you want to download" + ' ' + " " + filename + "" + ' ') .setPositiveButton("Yes", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { DownloadManager.Request request = new DownloadManager.Request(Uri.parse(url)); String cookie = CookieManager.getInstance().getCookie(url); request.addRequestHeader("Cookie", cookie); request.addRequestHeader("User-Agent", userAgent); request.allowScanningByMediaScanner(); request.setNotificationVisibility(DownloadManager.Request.VISIBILITY_VISIBLE_NOTIFY_COMPLETED); DownloadManager manager = (DownloadManager) getSystemService(DOWNLOAD_SERVICE); request.setDestinationInExternalPublicDir(Environment.DIRECTORY_DOWNLOADS, filename); manager.enqueue(request); } }) .setNegativeButton("No", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { dialogInterface.cancel(); } }) .show(); } private void checkPermission() { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) { if (shouldShowRequestPermissionRationale(Manifest.permission.WRITE_EXTERNAL_STORAGE)) { //show dialog AlertDialog.Builder builder = new AlertDialog.Builder(mActivity); builder.setMessage("Write External Storage Permission is Required"); builder.setTitle("Please Grant Permission"); builder.setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_CODE ); } }); builder.setNegativeButton("Cancel", null); AlertDialog dialog = builder.create(); dialog.show(); } else { //request permission ActivityCompat.requestPermissions( mActivity, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, MY_PERMISSION_CODE ); } } } } private class MyWebViewClient extends WebViewClient { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { if (Uri.parse(url).getHost().equals("www.irmone.com")) { //Content urls open in app return false; } else { //Open external links in external browser or apps Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url)); startActivity(intent); return true; } } } public class MyWebClient extends WebChromeClient { private View mCustomView; private WebChromeClient.CustomViewCallback mCustomViewCallback; protected FrameLayout mFullscreenContainer; private int mOriginalOrientation; private int mOriginalSystemUiVisibility; public MyWebClient() {} public Bitmap getDefaultVideoPoster() { if (MainActivity.this == null) { return null; } return BitmapFactory.decodeResource(MainActivity.this.getApplicationContext().getResources(), 2130837573); } public void onHideCustomView() { ((FrameLayout)MainActivity.this.getWindow().getDecorView()).removeView(this.mCustomView); this.mCustomView = null; MainActivity.this.getWindow().getDecorView().setSystemUiVisibility(this.mOriginalSystemUiVisibility); MainActivity.this.setRequestedOrientation(this.mOriginalOrientation); this.mCustomViewCallback.onCustomViewHidden(); this.mCustomViewCallback = null; } public void onShowCustomView(View paramView, WebChromeClient.CustomViewCallback paramCustomViewCallback) { if (this.mCustomView != null) { onHideCustomView(); return; } this.mCustomView = paramView; this.mOriginalSystemUiVisibility = MainActivity.this.getWindow().getDecorView().getSystemUiVisibility(); this.mOriginalOrientation = MainActivity.this.getRequestedOrientation(); this.mCustomViewCallback = paramCustomViewCallback; ((FrameLayout)MainActivity.this.getWindow().getDecorView()).addView(this.mCustomView, new FrameLayout.LayoutParams(-1, -1)); MainActivity.this.getWindow().getDecorView().setSystemUiVisibility(3846); } } public void onBackPressed() { if (myWebView.canGoBack()) { myWebView.goBack(); } else if (doubleTap) { super.onBackPressed(); } else { Toast toast = Toast.makeText(this, "Press Again To Exit", Toast.LENGTH_SHORT); toast.setGravity(Gravity.CENTER | Gravity.BOTTOM, 0, 0); toast.show(); doubleTap = true; Handler handler = new Handler(); handler.postDelayed(new Runnable() { @Override public void run() { doubleTap = false; } }, 2000); } } }
If you have any problems please comment below. And also don’t forget to share your ideas with us!!